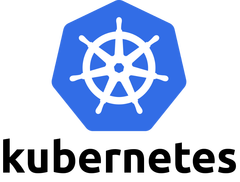
Kubernetes Cheatsheet
A comprehensive reference for Kubernetes (kubectl) commands, options, and examples.
Version: 1.28.0
Basic Commands
Essential commands for working with Kubernetes resources
kubectl get
Display one or many resources.
Examples:
kubectl get pods
kubectl get pods -o wide
kubectl get pods -A
kubectl get deployment,svc
kubectl describe
Show detailed information about a specific resource.
Examples:
kubectl describe pod my-pod
kubectl describe node my-node
kubectl describe deployment my-deployment -n my-namespace
kubectl create
Create a resource from a file or from stdin.
Examples:
kubectl create -f deployment.yaml
kubectl create namespace my-namespace
kubectl create deployment nginx --image=nginx
kubectl apply
Apply a configuration to a resource by file or stdin.
Examples:
kubectl apply -f deployment.yaml
kubectl apply -f https://raw.githubusercontent.com/kubernetes/examples/master/guestbook/frontend-deployment.yaml
kubectl apply -f ./dir
Pod Management
Commands for managing Kubernetes pods
kubectl run
Create and run a particular image in a pod.
Examples:
kubectl run nginx --image=nginx
kubectl run -it busybox --image=busybox -- sh
kubectl run nginx --image=nginx --port=80 --expose
kubectl exec
Execute a command in a container.
Examples:
kubectl exec my-pod -- ls /
kubectl exec -it my-pod -- bash
kubectl exec -it my-pod -c my-container -- bash
kubectl logs
Print the logs for a container in a pod.
Examples:
kubectl logs my-pod
kubectl logs -f my-pod
kubectl logs -p my-pod -c my-container
kubectl delete pod
Delete a pod.
Examples:
kubectl delete pod my-pod
kubectl delete pod my-pod --force --grace-period=0
kubectl delete pod -l app=nginx
Deployment Management
Commands for managing Kubernetes deployments
kubectl rollout
Manage the rollout of a resource.
Examples:
kubectl rollout status deployment/nginx-deployment
kubectl rollout history deployment/nginx-deployment
kubectl rollout undo deployment/nginx-deployment
kubectl rollout undo deployment/nginx-deployment --to-revision=2
kubectl scale
Set a new size for a Deployment, ReplicaSet, or Replication Controller.
Examples:
kubectl scale deployment nginx-deployment --replicas=3
kubectl scale --replicas=3 -f deployment.yaml
kubectl set image
Update image of a pod template.
Examples:
kubectl set image deployment/nginx-deployment nginx=nginx:1.16.1
kubectl set image deployment/nginx-deployment nginx=nginx:1.17.0 --record
Configuration
Commands for managing Kubernetes configuration
kubectl config
Modify kubeconfig files.
Examples:
kubectl config view
kubectl config current-context
kubectl config use-context my-cluster-name
kubectl config set-context --current --namespace=my-namespace
kubectl config get-contexts
Display one or many contexts from the kubeconfig file.
Examples:
kubectl config get-contexts
kubectl config get-contexts my-context